Configure Options – reading settings from appSettings – Dependency Injection – .NET CORE PART IV
Hi mates! How are you ? In this post, we are continuing with the dependency injection series, particularly, I’m talking about Configure Option and how we can interact with the appSettings.json.
If you didn’t see previous posts, you can check out:
- Dependency Injection Net Core – Part 1 – What is DI ? Applying DI in a Console Core Project
- Dependency Injection Net Core – Part 2 – Applying DI in a ASP.NET CORE Project
- Dependency Injection Net Core – Part 3 – Applying Extensions methods to register services – Clean Code
Ok, Let’s code π
If you remember, we have a SchoolService with a method GetPublicKey(). In this method, we have a TO-DO that says “retrieve to the config“.
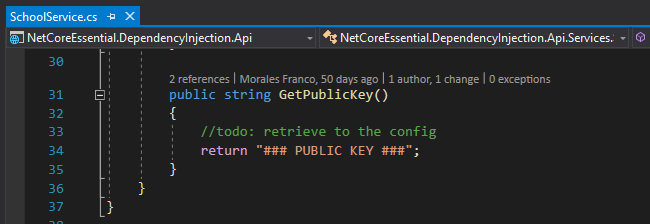
Retrieving an easy & simple key π
Imagine that you have this key:
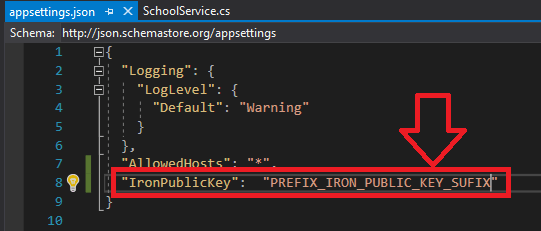
This key is not hierarchical, it is simple. For this, reason we can inject IConfiguration in our service to retrieve this value:
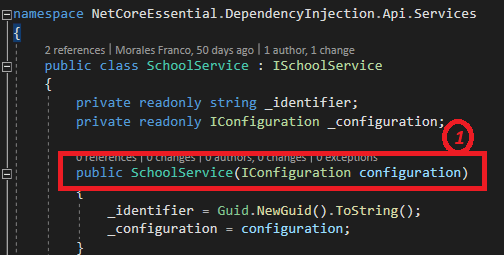
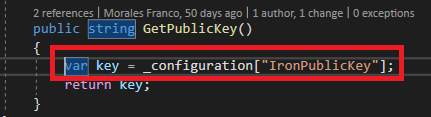
Let’s test that;) We’re gonna use POSTMAN for testing our Method and in addition, we’re gonna put a break-point in the method to check if it retrieves the config value successfully.
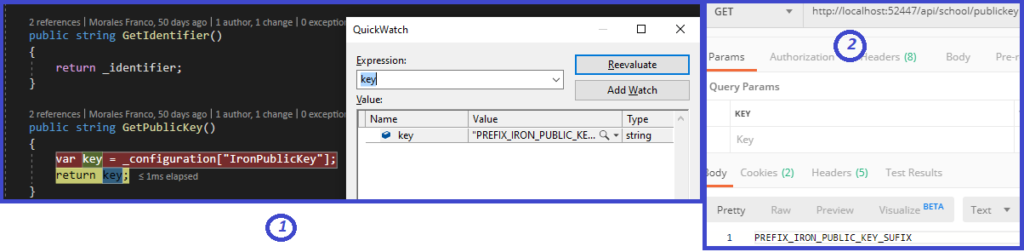
Great π we could retrieve the IronPublicKey successfully π
Complex Key with IConfiguration π
Right now, you imagine that you have a complex key in the appSettings, maybe you can have a hierarchical key with several keys nested such as:
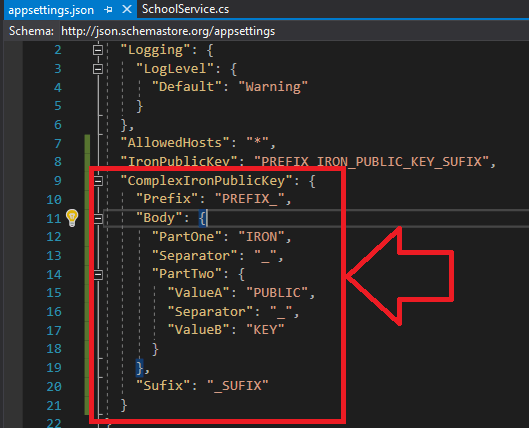
You can see our new ComplexIronPublicKey is hierarchical and a little more complex than IronPublicKey.
You can see three sections: Prefix, Body and Sufix.
We need to return the same value to the API but we have to do some logic before. We can think to use our friend IConfiguration to retrieve this key and maybe we can do something how this:
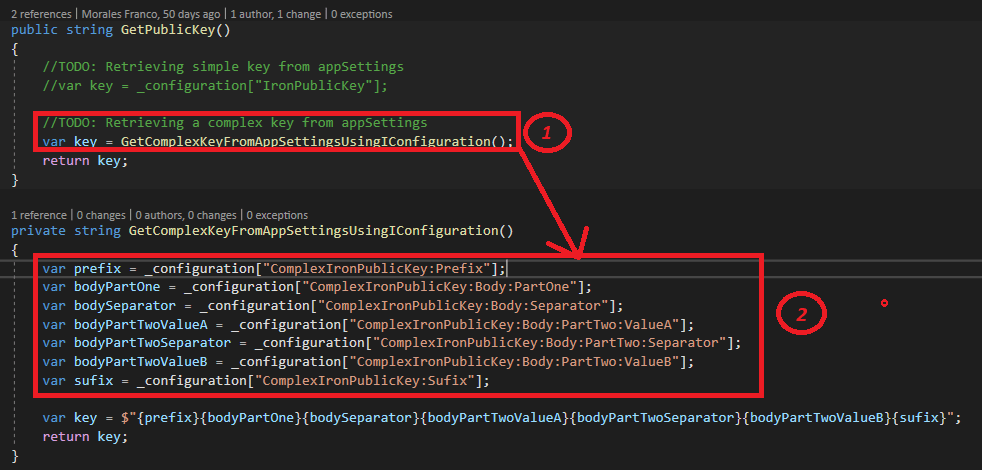
You can see that our method is a little complex but it works. We could retrieve each section from the appSettings and finally, we join every part that composes the ComplexIronPublicKey.
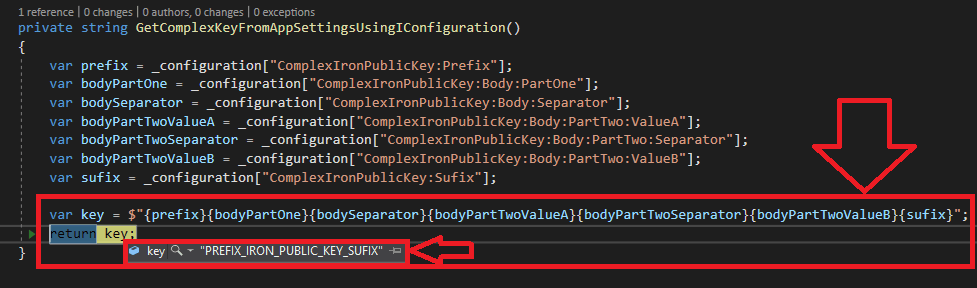
It works, we could to retrieve each value and make the IronPublicKey but Can you imagine if you need to use this value in several services? You would need to replicate this logic in each service, or of course, you might have a Helper and centralize all logic inside it. However, why not do we use dependency injection for that?
Retrieving a complex key using IOptions π
First, we need to create a class that will represent our complex key, in other words, a DTO. Then, we’ll configure this class in our Dependency Injection Engine and Finally, we’ll be able to inject this class wherever we want to use it.
Step 1: Creating DTO Configuration Class
This class need to represent our complex configuration section. In this case we have a hierarchical key, for this reason, we need to create a class with nested classes.
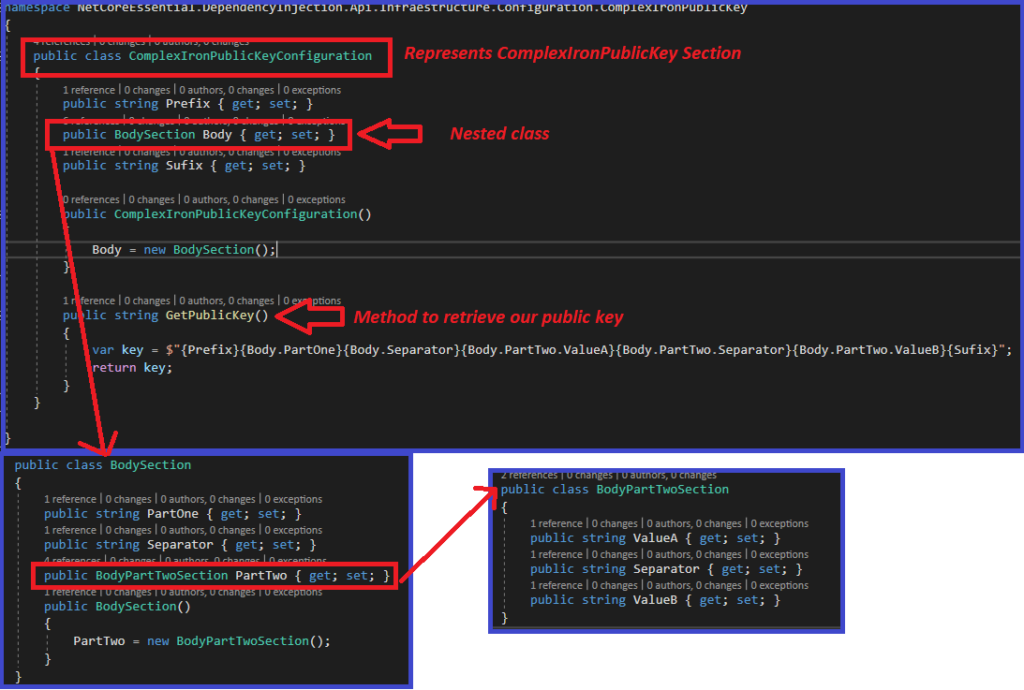
Step 2: Registering our Configuration class in the dependency injection engine
We need to register our class in the container, for this reason, we need to go to the ConfigureServices() method in the startup class.

Basically, we have to say to the container: “Ey, I have a ComplexIronPublicKeyConfiguration class that represents the ComplexIronPublicKey section in the appSettings file. Please, when I inject this key in a service please give me an instance of this class and filling it with the section values! “
Step 3: Injecting class in a service
Finally, we need to inject this class in a service where we want to use it π
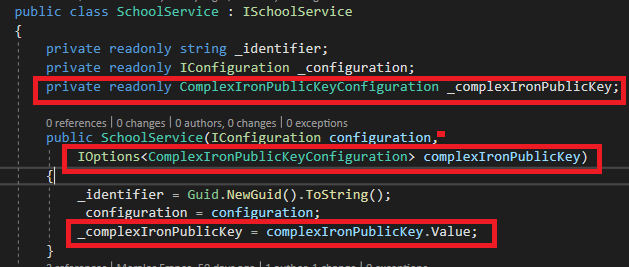
Important: you can see that when we inject IOptions<> then in the constructor, we use the property Value. Why do we need to do that ? Easy man, what happens if you change any value in the section ? Exactly if you wouldn’t use this property, the framework wouldn’t be able to read the new values.
The container doesn’t create N instances of our configuration class, only it fills our configuration class with the updated data.
If we inject this class in many services, we have the same instance as a singleton with the same hashCode.
Step 4: Let’s test π
We send a request to test this service π
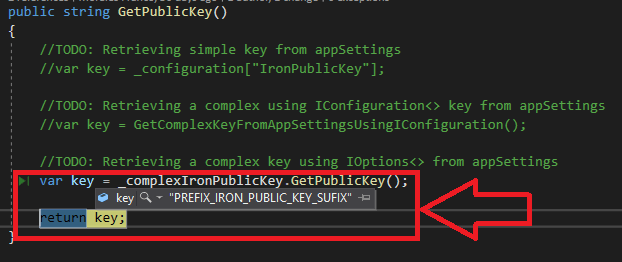
You can see how we were able to use our class to retrieve our complex key π only we need to call our GetPublicKey method.
Bonus clean code in ConfigurationService – Extension Method π
Of course, right now, we put the focus on good practices, for this reason, we will create an extension method to register every Configuration Service.
First, we need to create our extension method:
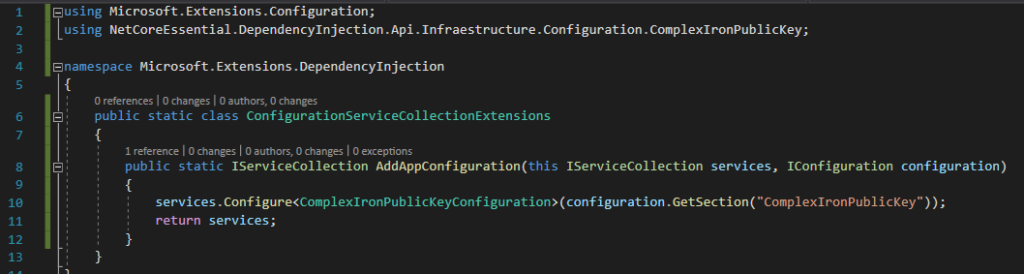
Second, we need to use our extension method in the ConfigureService method.
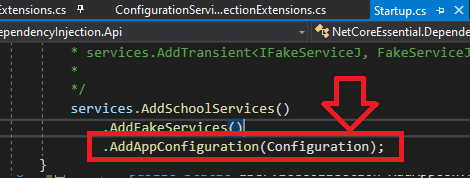
Furthermore, you can see that in this extension method, we need to pass the Configuration variable as a parameter (IConfiguration).
Summary
Ok, guys, we were able to use IOption feature to inject a complex setting. In this way, we can inject this setting inside classes that want to use it.
We can achieve to get a more scalable and maintainable code.
That’s all guys! I hope you enjoyed this post π
You can see this code on my github
Big hug !