Dependency Injection – .NET CORE PART II
Topics:
- Resume of Part I – Dependency Injection & dotnet core
- Using dependency injection in asp.net core project
Resume of part I
Dependency injection pattern (DI) is a technique to achieve: Inversion of Control (IoC).
For us: Dependency Injection container = Inversion of control Container.
DI will help us to simplify the management of all our dependencies.
Services are registered in the net core container specifically in the Startup class.
The container is responsible for creating and disposing of all service instances or in other words, it’s responsible for the life-time of all services registering in.
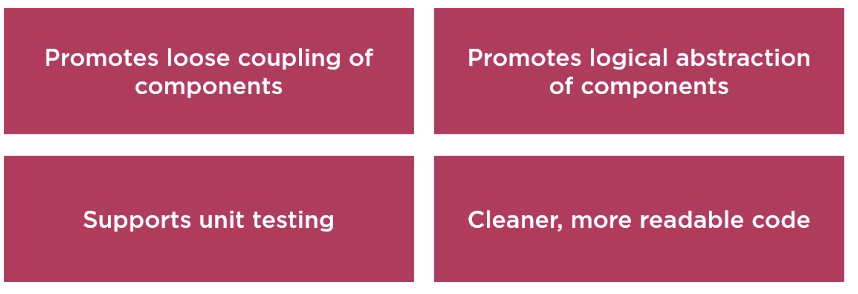
We have two key components:
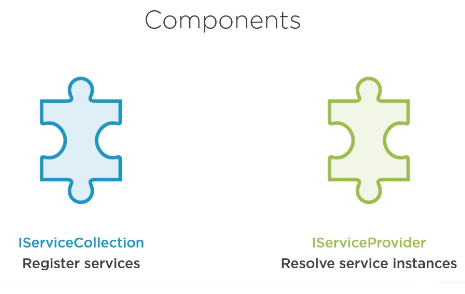
- IServiceCollection: our container – We need to register the services here.
All services need to be registering in the IServiceCollection (container) - IServiceProvider: we used this component to retrieve a requested service in runtime.
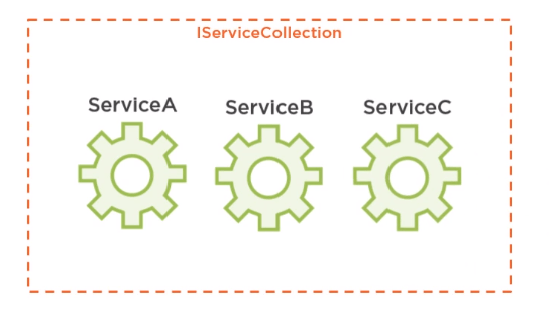
Ok enough theory for us 😉 Let’s code!
We have an asp.net core project empty and we will two new services:
- StudentService
- SchoolService
- BestTeacherService: We only have one teacher –> Singleton
Creating Services
First, we will create interfaces for concrete services. In this case, we will have:
- IStudentService (interface) –> StudentService (concrete)
- ISchoolService (interface) –> SchoolService (concrete)
- IBestTeacher (interface) –> BestTeacherService (concrete)
Generating interfaces:
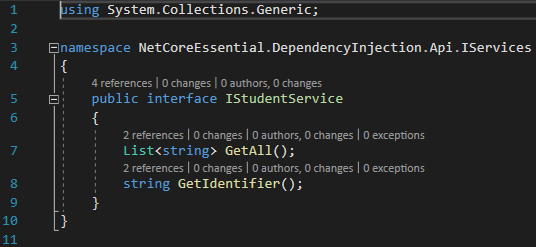
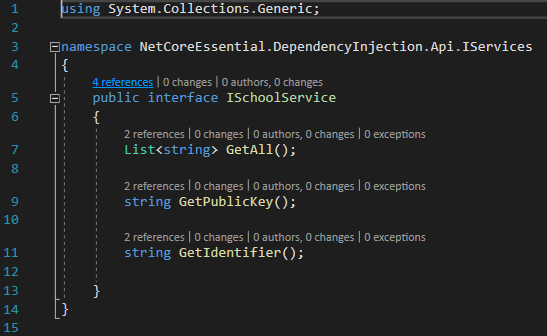
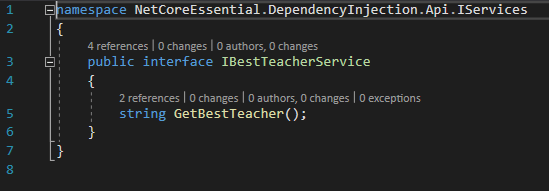
Then, we need to generate concrete classes which implement their interfaces:
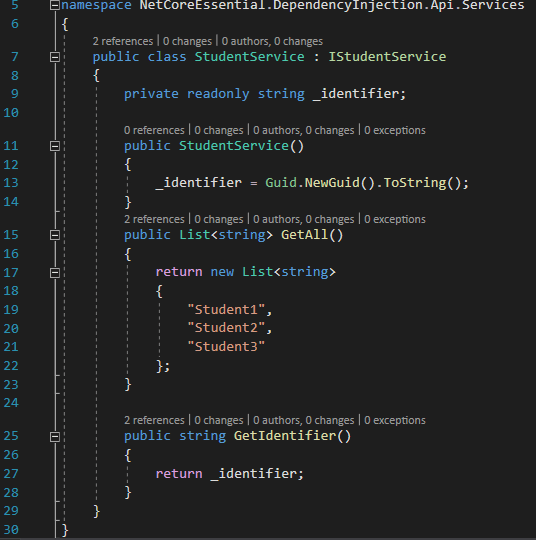
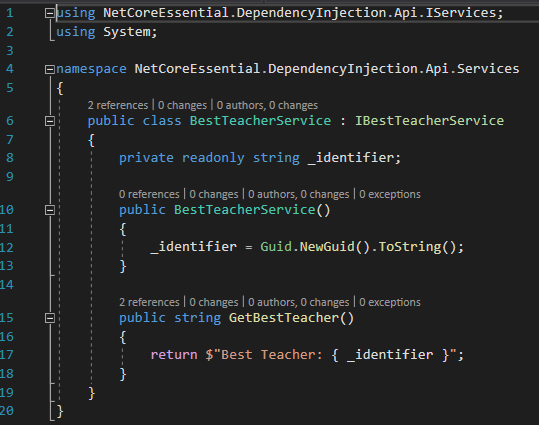
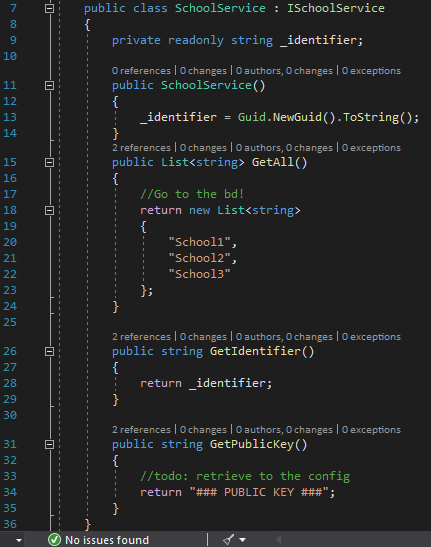
Important data: you need to put the focus in the IDENTIFIER. All the classes have un GUID as UNIQUE IDENTIFIER. This is initialized when each class is instantiated.
This will be important when we will test our system.
Perfect, we created all services and interfaces 😉
Registering services
We need to go to the startup.cs and register these services in the container. In this case, we will use a scoped lifetime for the school and student services because we want to get a new instance per request. However, the BestTeacherService will be a singleton.
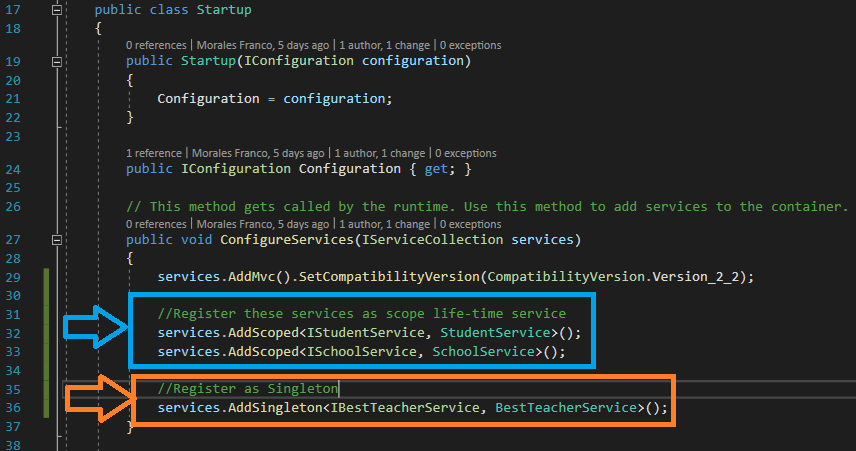
Injecting services in controllers
Finally, we need to create two API controllers:
- StudentController: this need to interact with StudentService
- SchoolController: this need to interact with SchoolService and bestTeacherService.
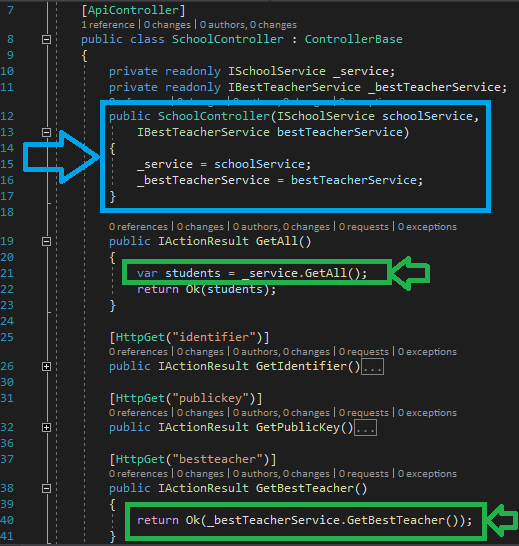
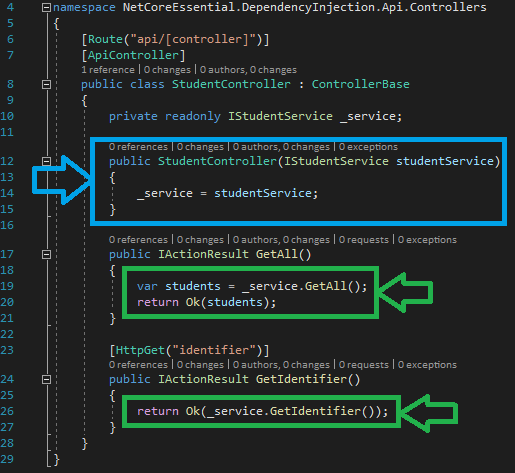
We have to put the focus on the methods which return the Identifier because these are UNIQUES depending on the lifetime of the services.
In other words:
- IStudentService & ISchoolService: are SCOPE Services. In each request must return a different identifier because the framework will create a new instance per request.
- IBestTeacherService: is SINGLETON. It will always return the same instance.
Testing School controller
api/student [GET] – (Get all)
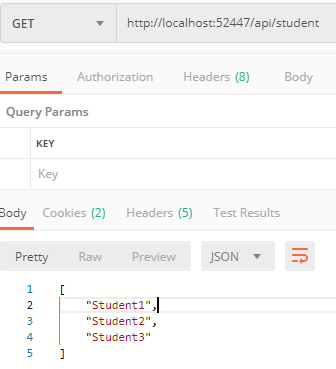
api/student/identifier [GET] – First time
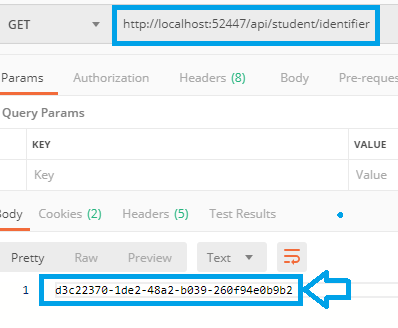
api/student/identifier [GET] – Second time
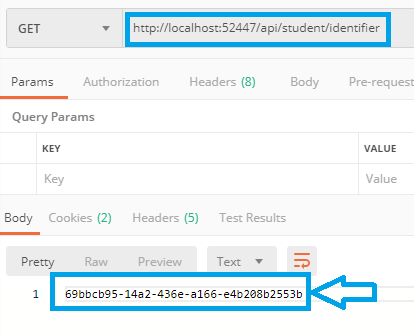
(*) You can see that the requests return different GUID’s because of the IStudentService is a SCOPE Service and the container create a new instance per request.
api/school/identifier [GET] – First time
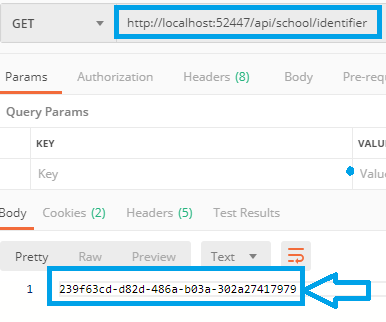
api/school/identifier [GET] – Second time
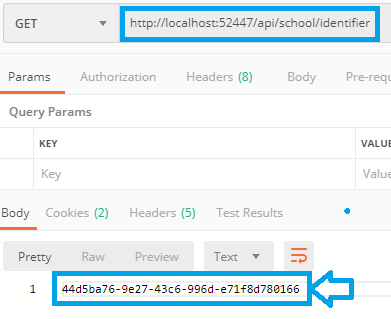
(*) ISchoolService has a SCOPE timelife, for this reason, the framework create a new instance, with different ID, per request.
/school/ bestteacher [GET] – first time
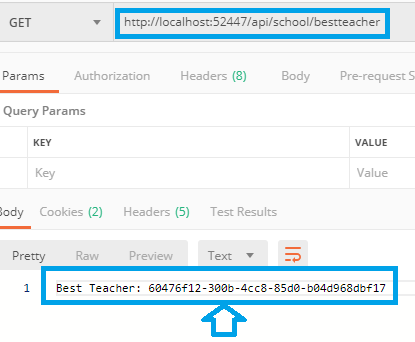
/school/bestteacher[GET] – second time
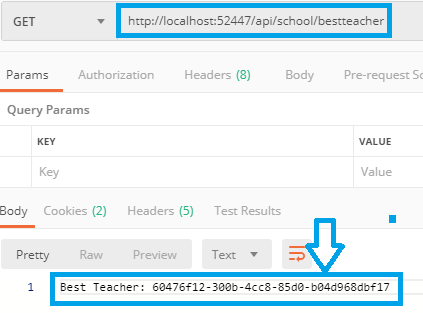
Magic in the case of BestTeacher, the controller always returns the same instance with the same ID because of the IBestTeacherService was registered as SINGLETON.
Ok perfect, it was enough for today 😀
In the next post of dependency injection, we will enjoy with other DI features 😉
Code on GitHub
Big hug!
3 thoughts on “Dependency Injection – .NET CORE PART II”
Leave a Reply
You must be logged in to post a comment.
Great article about Dependency Injection! Very simple and understandable. Happy coding !
Thanks very much ! I’m happy that you met useful this article!
That is the best blog for anyone who wants to search out out about this topic. You realize so much its nearly exhausting to argue with you (not that I really would want…HaHa). You positively put a brand new spin on a topic thats been written about for years. Great stuff, just great!