c# Datetime Examples
Datetime Class in c# is very useful. Now, we try to do many examples.
Let’s begin!
What does Datetime.now return?
When we code Datetime.Now, this return the current time but where does the framework take this data ?
Ok, we have many answers for it. For example :
*** In a web server, It takes the current time from the server.
*** In localhost, It takes the current time from the Operating System
Let me show you an example
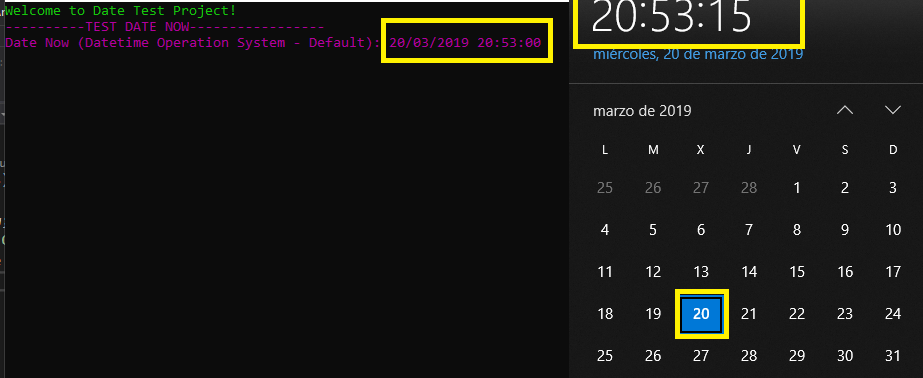
Now, we’ll proceed to change date & time in the OS. New time:
23 march 2022 – 12:05. After this change, we’ll execute Datetime.Now again:
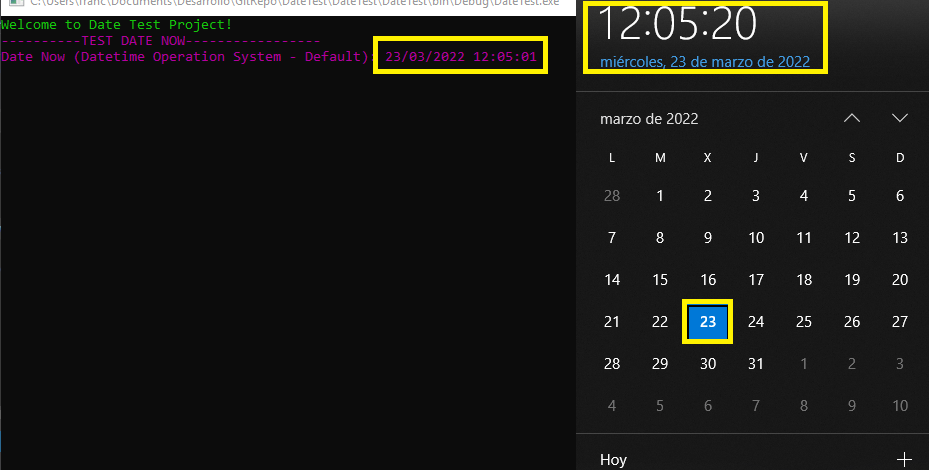
ToString (IFormatProvider provider)
Display datetime with specific culture:

CurrentCulture in the App!
You can check the current Culture in your app. You only need to consult to the Current Thread.
Thread.CurrentThread.CurrentCulture
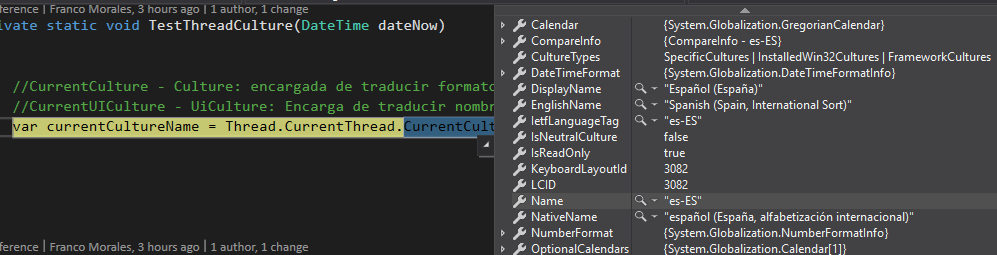
This show the current culture from the application. In my case es-ES but if I changed the time region from another country, the currentCulture would change too. The current Thread manage Date format, currency, etc
Ok, Now, We’ll try to change de current Thread Culture:
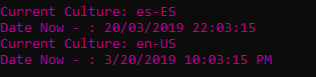
string to Datetime!
Many times we need to cast a string value to a Datetime. Be careful, mates!
In this example we need to cast “20/11/2019 22:09” to Datetime! Let’s code!
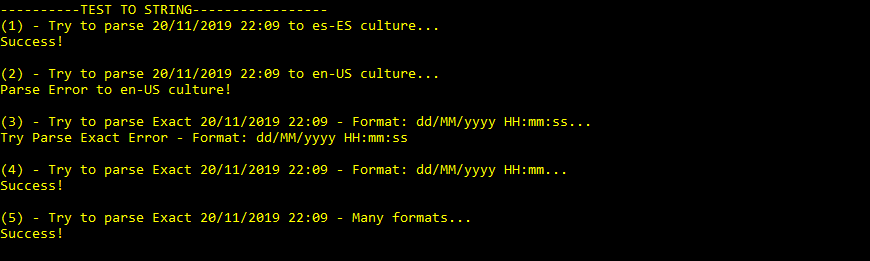
- Try to parse 20/11/2019 22:09 to es-ES culture –> Success ! Because in Spain culture the default format is: dd/MM/yyyy HH:mm:ss
- Try to parse 20/11/2019 22:09 to en-US culture –> Failed! Because in USA the default format is MM/dd/yyyy for this reason when we try to parse “20/11/2019” to Datetime, the framework assumes that the number 20 is the month but can the number 20 be a month? NO! EXCEPTION!
- Try to parse Exact 20/11/2019 22:09 – Format: dd/MM/yyyy HH:mm:ss… –> Failed! Because the string value doesn’t have seconds! It only has minutes! –> Failed!
- Try to parse Exact 20/11/2019 22:09 – Format: dd/MM/yyyy HH:mm… –> Success!
- Try to parse Exact 20/11/2019 22:09 – Many formats… –> We pass many allowed formats to the method! If some format matches with the value, success!
Convert String To Datetime – Convert() Method
Convert() is other alternative from cast a string to Datetime.
Let’s begin!
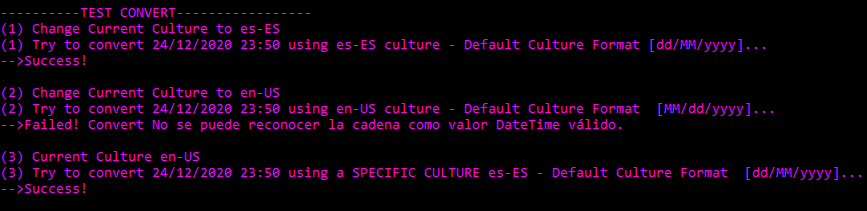
In this test you can show how we can use the Convert method to cast the string: “24/12/2020 23:50” to Datetime Object.
In this process is important the current culture because the framework use it to try to convert the string into a object (default behaviour) but you can a specify culture too.
Let’s analyze the sample:
- Current culture is ES – the default format for it, it’s : “dd/MM/yyyy”. When we try to convert “24/12/2020 23:50” to this culture, it’s valid! Success!
- Now the Current culture is US – the default format for it, it’s : “MM/dd/yyyy”. When we try to convert “24/12/2020 23:50” to this culture, we can see that the month would be 24 for the framework! Failed! Exception!
- In this step the Current Culture is US – the default format for it, it’s : “MM/dd/yyyy”. The default Convert() method’ll fail when we’ll try to convert “24/12/2020 23:50” to this culture (we could see that in the previous step)! For this reason, we’re specifying a CULTURE ES because for this culture the default format is: “dd/MM/yyyy” and the convert method’ll be successfull!
That’s all!
Complete example on Github: https://github.com/morales-franco/DateTest
Regards!
F.M
Regards!
F.M
1 thought on “c# Datetime Examples”
Leave a Reply
You must be logged in to post a comment.
Someone essentially assist to make significantly posts I’d state. That is the first time I frequented your web page and to this point? I amazed with the analysis you made to create this particular post amazing. Fantastic job!