Design Patterns – Singleton
Singleton is a creational design pattern.
Idea: We want to have some classes that should have EXACTLY ONE INSTANCE.
Singleton Pattern ensures a class has only one Instance.
Uses:
- When creating the instance is expensive –> a Singleton can improve performance.
- There must be one and only one instance of a class.
- The class should not require parameters as a part its construction.
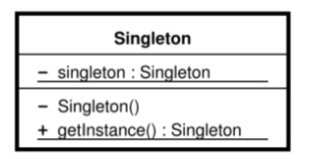
The image shows us a Singleton class in its classic version. This version has:
- Private static instance of Singleton class
- Private Constructor
- Public static Method which returns the UNIQUE singleton Instance.
Version 1 – Classic Singleton
This is the original version of the pattern. In other words, the Default Implementation.
This version is NOT THREAD-SAFE. For this reason should not be used in multi-threaded environments (E.g: Web Servers – ASP.NET)
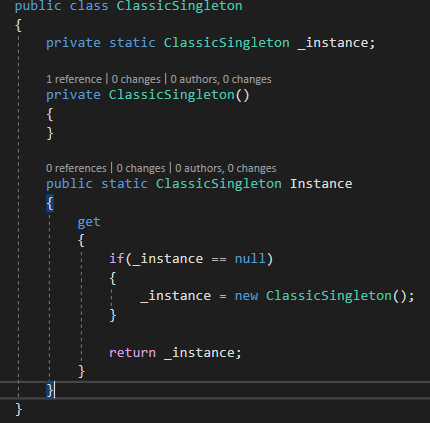
Version 2 – Thead-safe Singleton using double-check locking
This version is thead-safe but doesn’t perform as well as Lazy Implementation.
It’s easy to get wrong with this alternative –> A pattern should be more cleaning.
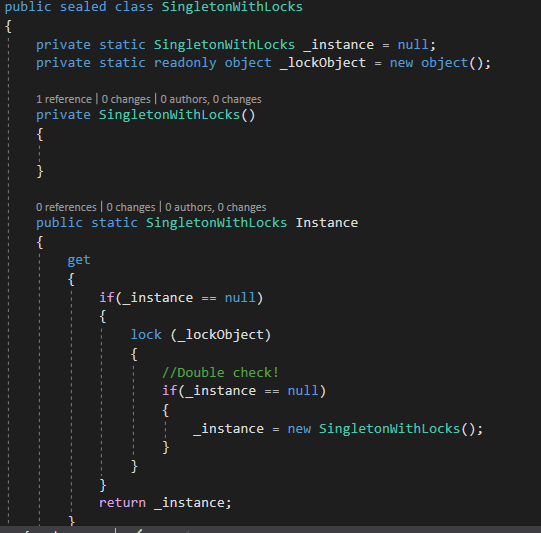
Version 3 – Recommended – Thead-safe Singleton with Lazy<>
- It’s simple and performs well
- By Default, Lazy objects are thread-safe
- We can use this alternative in web projects and multi-threaded environments.
Finally, I will call all Singletons!
static void Main(string[] args)
{
Console.WriteLine(ClassicSingleton.Instance.SayHello());
Console.WriteLine(SingletonWithLocks.Instance.SayHello());
Console.WriteLine(SingletonLazy.Instance.SayHello());
}